Client API
Sophora's Client API provides an interface for partners and customers who want to develop their own client applications. For the development process it is required to include subshell's external Maven repository.
Initialising an ISophoraClient
The most convenient way to initialize an ISophoraClient is to use the SophoraClientBuilder, which allows you to set up a Sophora Client with a variety of options including settings for proxy servers or caching options.
The following example shows how easy it is to create a client with the SophoraClientBuilder
.
ISophoraClient client = SophoraClientBuilder.newBuilder()
.withHost("https://my-sophora-server:1197")
.withCredentials("username", "password")
.login() // this will return a logged-in client, thus you won't have to call login() separately on the created client
.build();
// do something with the client
client.logout(); // the Sophora client also implements the Autoclosable interface
Note, that this is just an example. The builder offers many more methods to configure the Sophora Client, including the following and even more than those:
.withProxy(host, port)
.withProxyAuthentification(username, password)
.withSocksProxy(host, port)
.withNumberOfCachedDocuments(number)
.withMemoryCacheForMultipleLogins()
- This is necessary if more than one ISophoraClient is instantiated in the same JVM.withCacheDir(absolute path)
.withThumbnailCacheSize(size)
- and many more!
You may also initialise an ISophoraClient with multiple hosts. Just provide a list of strings instead of a simple string as the first parameter to SophoraClientBuilder.newBuilder()
.
Starting with the first host in the list, the Sophora Client will try all hosts until it connects successfully to the Sophora Server.
If you do not want the ISophoraClient to try to connect to any other previous seen hosts, use the .withNoOtherHosts()
option. (Previous connections are cached in a file in the Sophora home directory).
Initialising an ISophoraClient and adding a listener (here an IDocumentChangeListener
), but you can add any implementation of IContentListener
, IDocumentChangeListener
, ContentAdapter
etc.
try(ISophoraClient client = SophoraClientBuilder.newBuilder()
.withHost("https://my-sophora-server:1197")
.withCredentials("username", "password")
.login()
.build()) {
client.addListener((IDocumentChangeListener) dce -> {
System.out.println("document changed event: uuid: " + dce.getUUID() + ", primaryType: " + dce.getPrimaryType() + ", stateChange: " + dce.getStateChange());
});
}
Restrictions of Sophora's Client API
The current version of the Sophora Client API cannot handle multiple sessions in one Sophora Client. This requires that for each user logged in to Sophora an individual instance of the Sophora Client has to be created.
Document States and Transitions
The following diagram shows the basic document lifecycle.
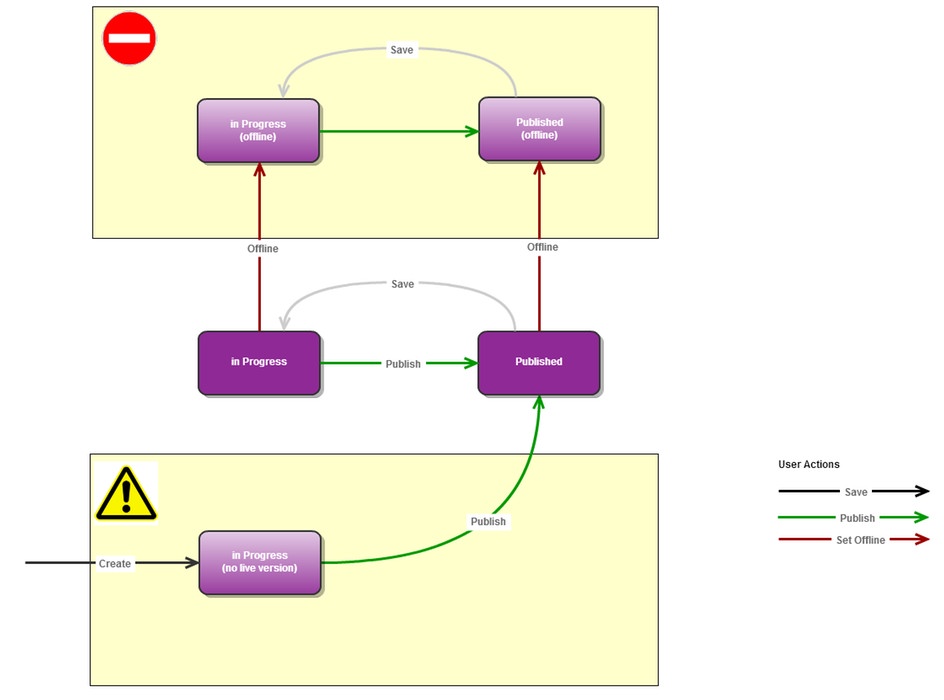
Depending on the configuration of your Sophora installation, additional states may be available for some document types.
The following diagram shows all possible transitions between document states, except for the "deleted" state.
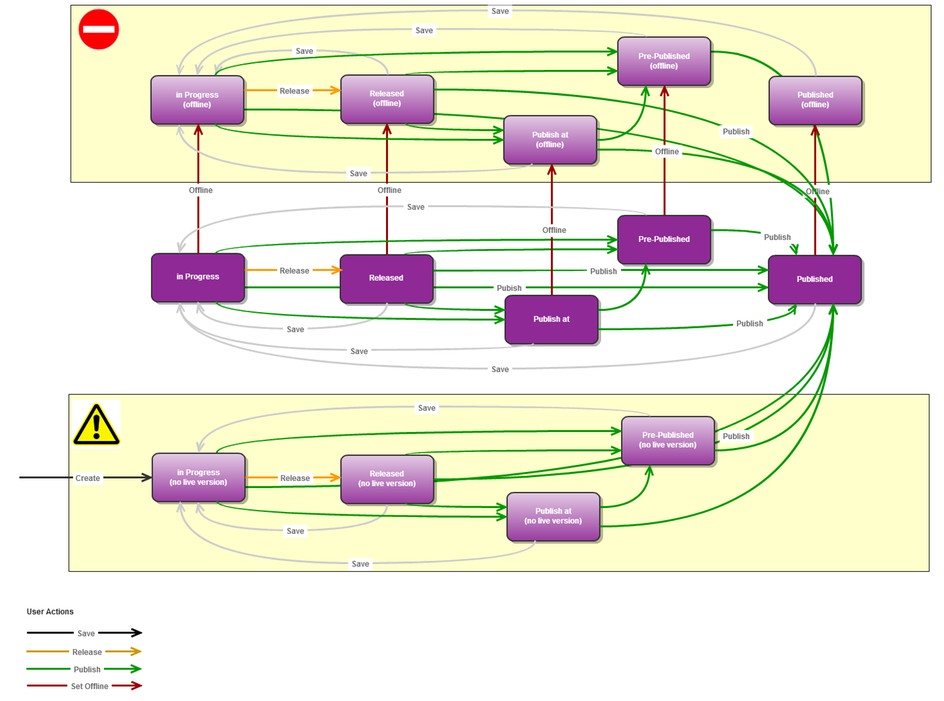
Publishing documents with version parameters
For furthers actions related to the publication of a document (e.g. adding certain properties automatically or pushing document specific information to 3rd party APIs like social media) it's useful to add metadata to the publication process that can be evaluated by scripts running in the Sophora server or a client or within tools that are receiving events from the server via the Sophora Client API.
This can be done by adding VersionParameters
when calling the publish
method, either from an ISopharaClient
instance or within a Sophora client or server script.
Example code for publishing a document with version parameters
UUID uuid = ... // given UUID
ISophoraClient client = ... // initialized ISophoraClient instance
// Create version parameters and call publishDocument()
VersionParameters versionParameters = VersionParameters.of("foo", "bar");
client.publishDocument(uuid, versionParameters);
Version parameter occurrence
The version parameters are visible:
- In events received by an event listener registered with
client.addListener()
- In events handled by state change scripts (see Example 4 on this page)
- In the document version history shown in the Sophora DeskClient
The version parameters passed to the publish
method are saved in the published version of the document, so that the version parameters for each publication of a document can be seen in the document's version history.
However it is important to retrieve the version parameters for the current publication process from the PUBLISH
event, because the version parameters are added to the document at the end of the publication process. Only the finally published version of the document contains the version parameters, the document passed to event handlers does not yet contain the version parameters property.
The version parameters will also be automatically removed from the document when the document will be saved again.
Example code to react on a publication with version parameters
The following code fragment creates a new IDocumentChangeListener
instance that checks for the version parameters foo
and bar
. The code is just a simple example and only writes log messages, but it can be extended to do something more useful:
[...]
Logger log = LoggerFactory.getLogger(this.getClass());
IDocumentChangeListener listener = (e) -> {
log.info("Got document change event: {}", e);
// Version parameters are only available for PUBLISH
if (e.getStateChange() != StateChange.PUBLISH) {
return;
}
if (hasVersionParameter(e, "foo")) {
log.info("published with versionParameter 'foo'");
// do something foo specific
}
if (hasVersionParameter(e, "bar")) {
log.info("published with versionParameter 'bar'");
// do something bar specific
}
};
ISophoraClient client = ... // initialized ISophoraClient instance
client.addListener(listener);
[...]
// Helper methode to check for a given version parameter
private static boolean hasVersionParameter(DocumentChangedEvent e, String name) {
return e.getVersionParameters()
.stream()
.anyMatch(v -> name.equals(v.getParameterName()));
}
Select value document for version parameters
To provide a user friendly representation of the version parameter names, there is a select value document named Versionsparameter
that provides a mapping for the internal name of the version parameter and a label that is shown to users.
In our example we use this mapping:
Key | Label |
---|---|
foo | Published with foo |
bar | Published with bar |
Predefined version parameters
In certain cases, Sophora applies version parameters automatically. The following table lists all of these parameters and under which circumstances they are applied.
In order for the corresponding name (instead of the technical value) to appear in the version history of the documents, the value/name pairs must be entered in the Versionsparameter
select value document as described above.
Parameter Name | Condition |
---|---|
publishMoved | When publishing documents in the course of a move, the moved document is published with the version parameter publishMoved ("Umverortet"). |
publishTransitive | When co-publishing components, the selected documents to be co-published are published with the version parameter "publishTransitive " ("Mitveröffentlicht"). |
Version history table in DeskClient
The labels of the version parameters from the select value document are shown in the version history for a Sophora document.
All publications create a new version of the document, the version parameters are shown for all published versions.
This is how the version history table looks like for a document published four time:
- with no version parameter
- with version parameter
foo
- with version parameter
bar
- with version parameters
foo
andbar
ID | Version created at | Parameters | Online from | User |
---|---|---|---|---|
test-100 | 2022-03-17 17:30:45 | Published with foo, Published with bar | 2022-03-17 17:25:16 | admin |
test-100 | 2022-03-17 17:25:16 | Published with bar | 2022-03-17 17:25:16 | admin |
test-100 | 2022-03-17 17:22:52 | Published with foo | 2022-03-17 17:22:52 | admin |
test-100 | 2022-03-17 17:21:19 | 2022-03-17 17:21:19 | admin |
External Maven Repository
To access our external Maven repository use the following address: "http://software.subshell.com/artifactory/repo-maven2". There, you'll find JARs and tools which partners and customers need for their own Sophora Client developments.
The libraries listed below are currently available:
- Sophora API
- Sophora Client
- Sophora Delivery
- Sophora Delivery-Content
- Sophora Commons
Within your project pom.xml
insert the following exemplary snippet:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"<br/> xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"><br/>
<modelVersion>4.0.0</modelVersion>
<br/>
<groupId>Insert your groupID here</groupId>
<br/>
<artifactId>Insert your artifactId here</artifactId>
<br/>
<version>Insert the according version here</version>
<br/>
<br/>
<repositories>
<br/>
<repository>
<br/>
<id>subshell</id>
<br/>
<url>https://software.subshell.com/artifactory/repo-maven2</url>
<br/>
</repository>
<br/>
</repositories>
<br/>
<br/>
<dependencies>
<br/>
<dependency>
<br/>
<groupId>com.subshell.sophora</groupId>
<br/>
<artifactId>com.subshell.sophora.client</artifactId>
<br/>
<version>4.0.0</version>
<br/>
</dependency>
<br/>
</dependencies>
<br/>
<br/>
</project>