Client API
Sophora's client API provides an interface for partners and customers who want to develop their own Sophora Client application(s).
For the development process it is recommended to include subshell's external Maven repository for Sophora Server tools.
Initialising an ISophoraClient
The most convenient way to initialize an ISophoraClient is to use the SophoraClientBuilder, which allows you to set up a Sophora Client with a variety of options including settings for proxy servers or caching options.
The following example shows how easy it is to create a client with the SophoraClientBuilder
.
ISophoraClient client = SophoraClientBuilder.newBuilder("https://my-sophora-server:1197", "username", "password")
.login() // this will return a logged-in client, thus you won't have to call login() separately on the created client
.build();
// do something with the client
client.logout();
Note, that this is just an example. The builder offers many more methods to configure the client, including the following and even more than those:
.withProxy(host, port)
.withProxyAuthentification(username, password)
.withSocksProxy(host, port)
.withNumberOfCachedDocuments(number)
.withMemoryCacheForMultipleLogins()
- This is necessary if more than one ISophoraClient is instantiated in the same JVM.withCacheDir(absolute path)
.withThumbnailCacheSize(size)
- and many more!
Initialising an ISophoraClient and adding a listener (here an IDocumentChangeListener
), but you can add any implementation of IContentListener
, IDocumentChangeListener
, ContentAdapter
etc.
ISophoraClient client = SophoraClientBuilder.newBuilder("https://my-sophora-server:1197", "username", "password")
.login()
.build();
client.addListener((IDocumentChangeListener) dce -> {
System.out.println("Handling document changed event: uuid: " + dce.getUUID() + ", primaryType: " + dce.getPrimaryType() + ", stateChange: " + dce.getStateChange());
});
Restrictions of Sophora's Client API
The current version of the Client API cannot handle multiple sessions in one client. This requires that for each user logged in to Sophora an individual instance of the Sophora Client has to be created.
Document States and Transitions
The following diagram shows the basic document lifecycle.
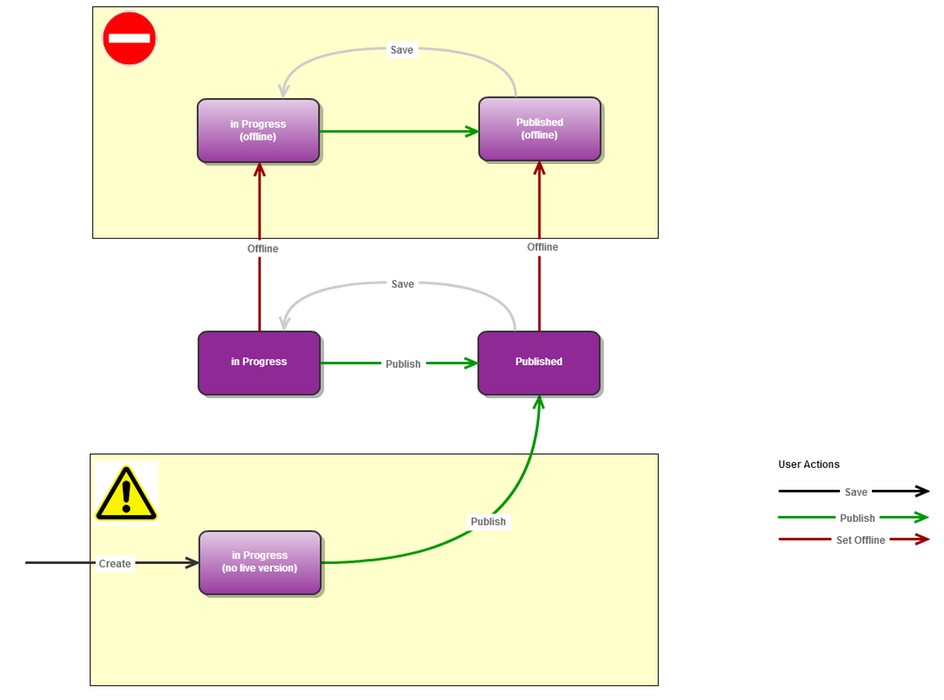
Depending on the configuration of your Sophora installation, additional states may be available for some document types.
The following diagram shows all possible transitions between document states, except for the "deleted" state.
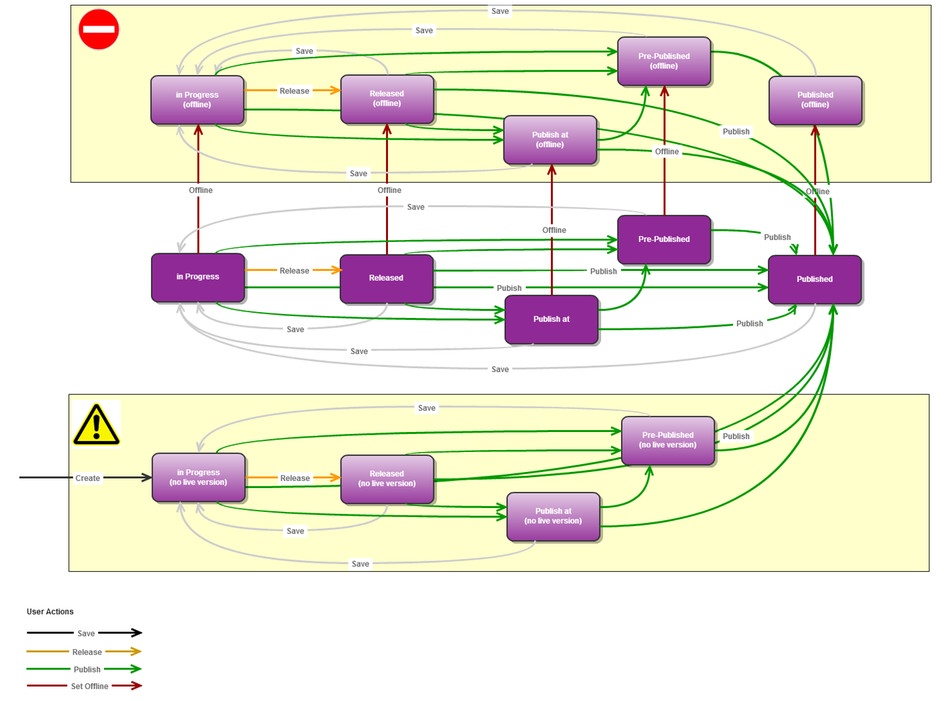
External Maven Repository
To access our external Maven repository use the following address: "http://software.subshell.com/artifactory/repo-maven2".
There, you'll find JARs and tools which partners and customers need for their own client developments.
The libraries listed below are currently available:
- Sophora API
- Sophora Client
- Sophora Delivery
- Sophora Delivery-Content
- Sophora Commons
Within your project pom.xml
insert the following exemplary snippet:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"<br/> xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"><br/>
<modelVersion>4.0.0</modelVersion>
<br/>
<groupId>Insert your groupID here</groupId>
<br/>
<artifactId>Insert your artifactId here</artifactId>
<br/>
<version>Insert the according version here</version>
<br/>
<br/>
<repositories>
<br/>
<repository>
<br/>
<id>subshell</id>
<br/>
<url>https://software.subshell.com/artifactory/repo-maven2</url>
<br/>
</repository>
<br/>
</repositories>
<br/>
<br/>
<dependencies>
<br/>
<dependency>
<br/>
<groupId>com.subshell.sophora</groupId>
<br/>
<artifactId>com.subshell.sophora.client</artifactId>
<br/>
<version>3.1.1</version>
<br/>
</dependency>
<br/>
</dependencies>
<br/>
<br/>
</project>